In the previous post we saw the limitations of using Option and in this post we are going to solve them choosing a different data type.
The issue with option was that when something goes wrong we have no information about the failure. We just know that our expected value is missing.
So we need a data type that holds some representation of the result of a failed execution. This data type is called Either.
Option was like a box that could contain a value, Either instead is a magic box that can contains a value of a type or another value of an other type.
By conventions those two possible values are called Left and Right, and usually we store the error in the left and the correct value in the Right.
Let’s use Either
Like we did for Option, we are going to use one Either to represent the mail, one Either to represent the phone number and then we will merge them to obtain the output of our validation
In our representation we will use Right to wrap valid input and Left to wrap invalid input, so for example:
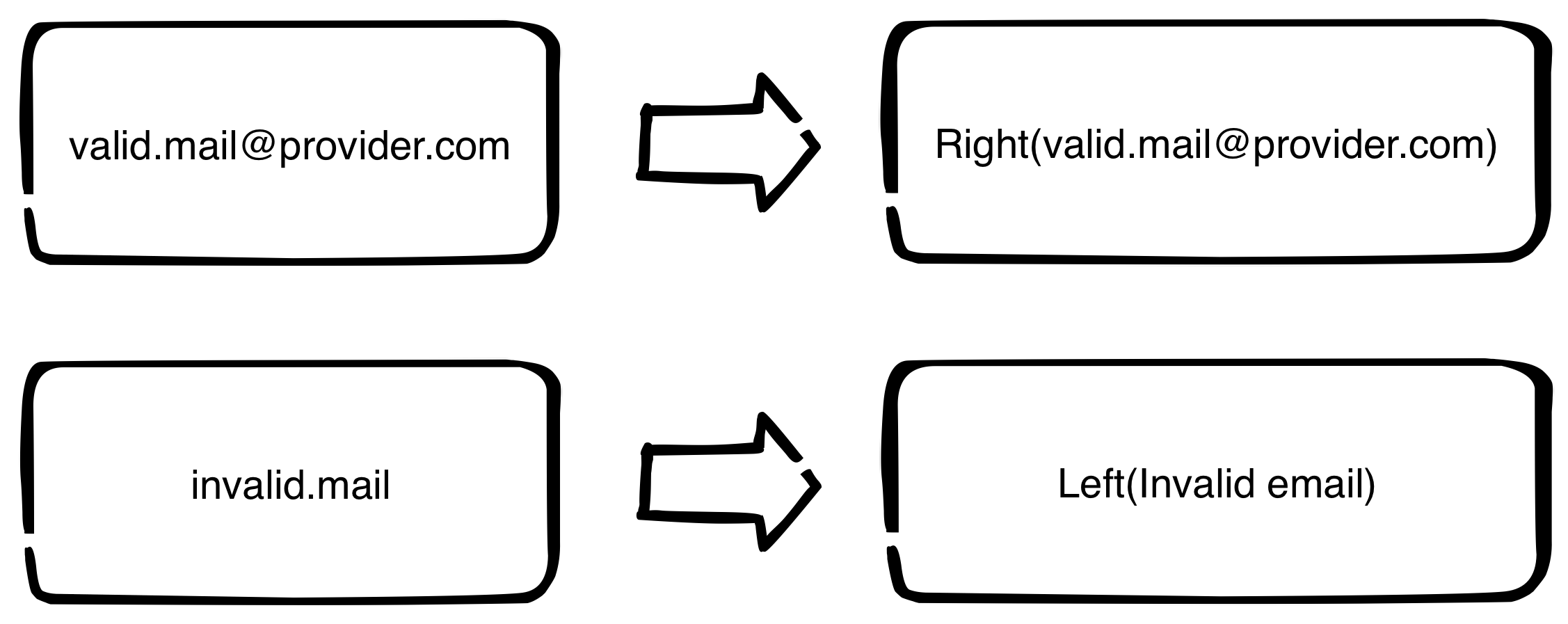
Once again to obtain such behaviour we can use kotlin extension methods invoking validMail and validNumber functions we have discussed in the first post
Map them
We have already discusse how map works in the previous post, so let’s use it:
The <String>
you are reading in the code is needed because we are using Strings to model errors.
Using valid mail and phone number the output will be:
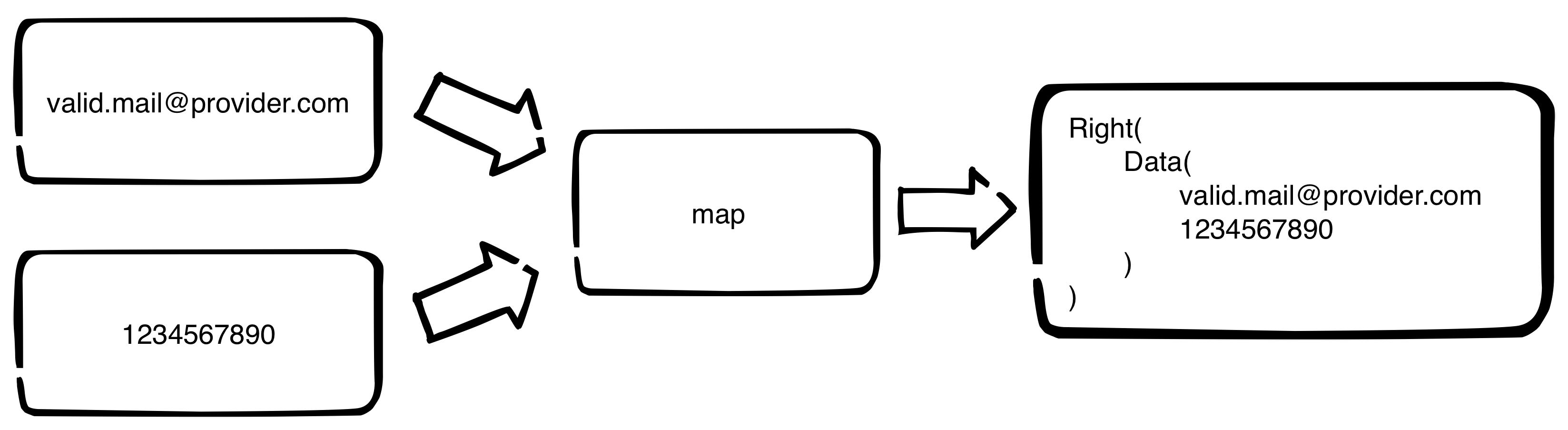
But the improvement compared with Option is that now we can also understand what goes wrong, so if we provide and invalid mail:
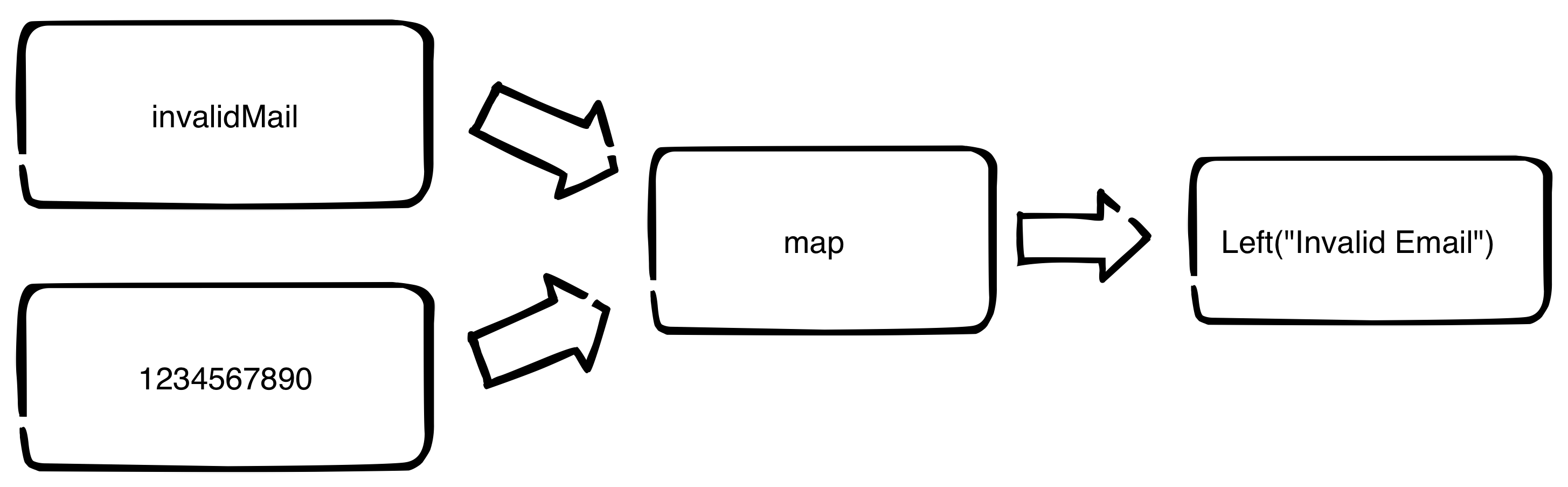
or an invalid phone number:
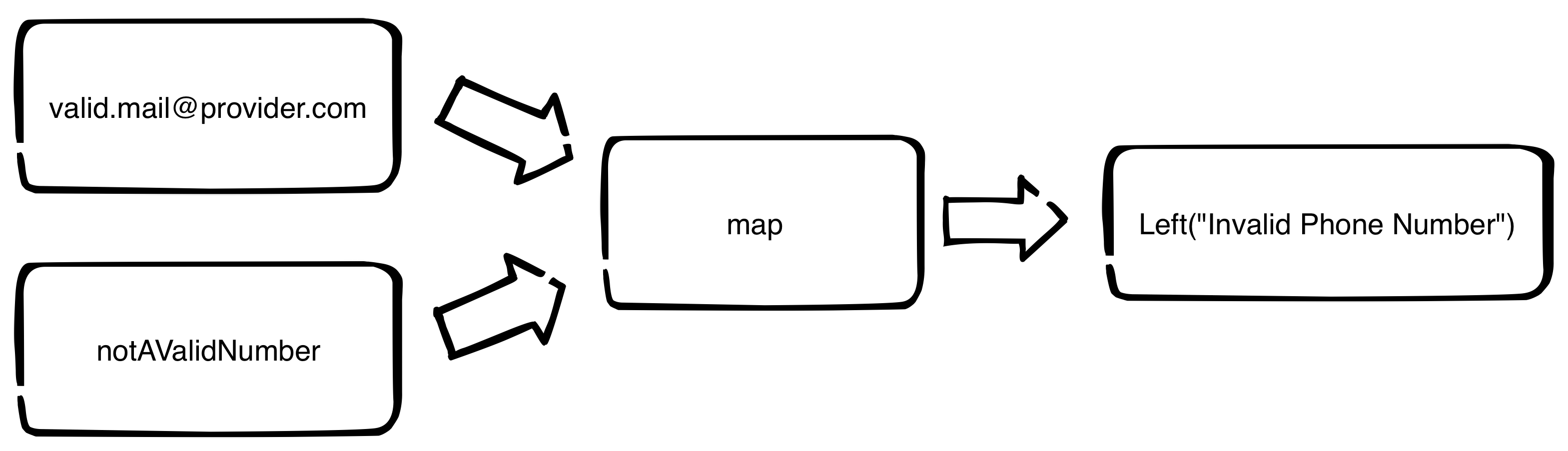
We finally have something to show to the user when he’s giving us wrong data!
Cool, but…
Either limits
What happens when all inputs are wrong?
Map implementation of Either will give us just the first error, we don’t have (yet) a way to accumulate errors.
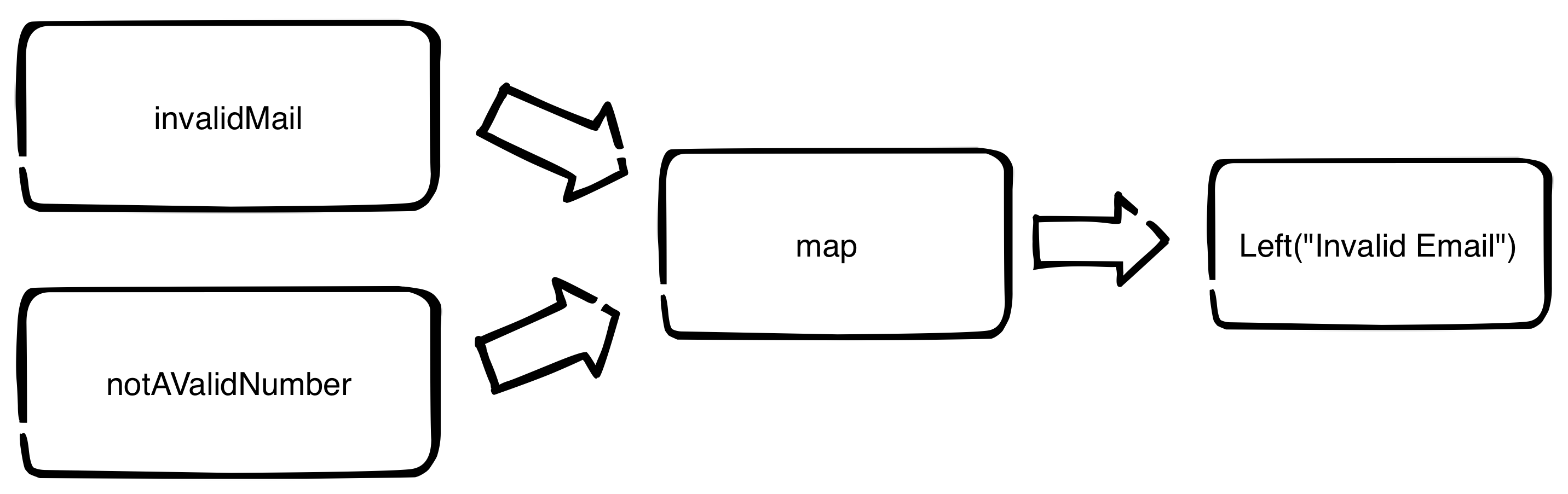
Android UI
Let’s update our android form to use Either.
In our ViewModel we will use the previously discussed code to update a LiveData<Either<String, Data>>
In the activity we retrieve the viewModel, register click listener and observe the result of the validation. Once we get the result we fold it.
Fold
Once again we are using method reference with the following methods:
This time we are getting an input in each method, in the first one we are getting a String that represents the error, while in the second we are getting the value of the Right, a Data instance in our implementation.
But, as previously discussed, if the user will give us multiple invalid inputs we will notify him just the first error, so Either is not a great choice for validation.
In the next post we will use our last data type that will solve all our problems!